WebAPI (Application Programming Interface) on üldnimetus kõigile API-dele, mida kasutatakse veebiteenuste vahel Interneti kaudu suhtlemiseks.
KML (Keyhole Markup Language) on failivorming, mida kasutatakse geograafiliste andmete kuvamiseks geobrauserites, nagu Google Earth, Google Maps ja Google Maps mobiilseadmetele.
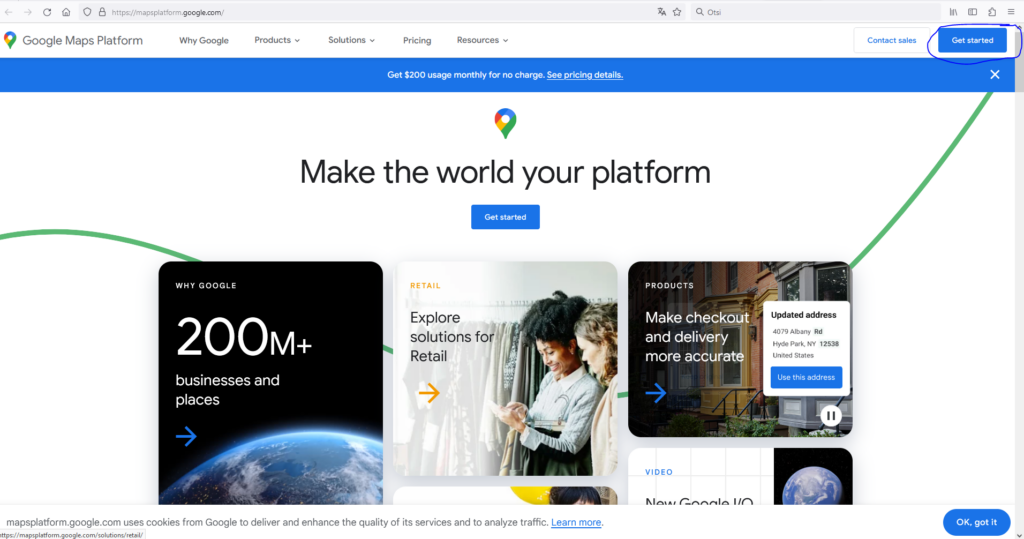
Registreeruge ja saate 300 tasuta dollarit. Järgmisena looge võti vastavalt juhistele
Index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Add Map</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<nav>
<ul>
<li>
<a href="../catapi/index.html">Cat API</a>
</li>
<li>
<a href="../apimap/index.html">Map API</a>
</li>
</ul>
</nav>
<h1>My Google Maps Demo</h1>
<div id="map"></div>
<script src="script.js" defer></script>
</body>
<footer>
<strong>© D.Miljukova</strong>
</footer>
</html>
Script.js
// Initialize and add the map
let map;
async function initMap() {
// Координаты для Retke tee 24
const position = { lat: 59.400859, lng: 24.705215 };
console.log("Initializing map at position:", position); // Отладка
// Request needed libraries.
const { Map } = await google.maps.importLibrary("maps");
const { AdvancedMarkerElement } = await google.maps.importLibrary("marker");
const map = new Map(document.getElementById("map"), {
center: { lat: 59.400859, lng: 24.705215 },
zoom: 18,
mapId: "4504f8b37365c3d0",
});
const marker = new AdvancedMarkerElement({
map,
position: { lat: 59.400859, lng: 24.705215 },
title: "Minu koju"
});
console.log("Marker added to the map.");
}
const g = { key: "AIzaSyD1NFUIIMc4zaMeKkVcg_ksaKEVGjnu7to", v: "weekly" };
(function(g) {
const script = document.createElement('script');
script.src = `https://maps.googleapis.com/maps/api/js?key=${g.key}&callback=initMap`;
script.async = true;
script.defer = true;
document.head.appendChild(script);
})({ key: "AIzaSyD1NFUIIMc4zaMeKkVcg_ksaKEVGjnu7to" });
Style.css
#map {
height: 80%;
}
html,
body {
height: 100%;
margin: 0;
padding: 0;
}
nav {
background-color: #007bff;
padding: 10px;
border-radius: 5px;
margin-bottom: 20px;
}
nav ul {
list-style: none;
padding: 0;
margin: 0;
display: flex;
justify-content: center;
}
nav li {
margin: 0 15px;
}
nav a {
color: white;
text-decoration: none;
font-weight: bold;
font-size: 16px;
transition: color 0.3s;
}
nav a:hover {
color: #d4d4d4;
}
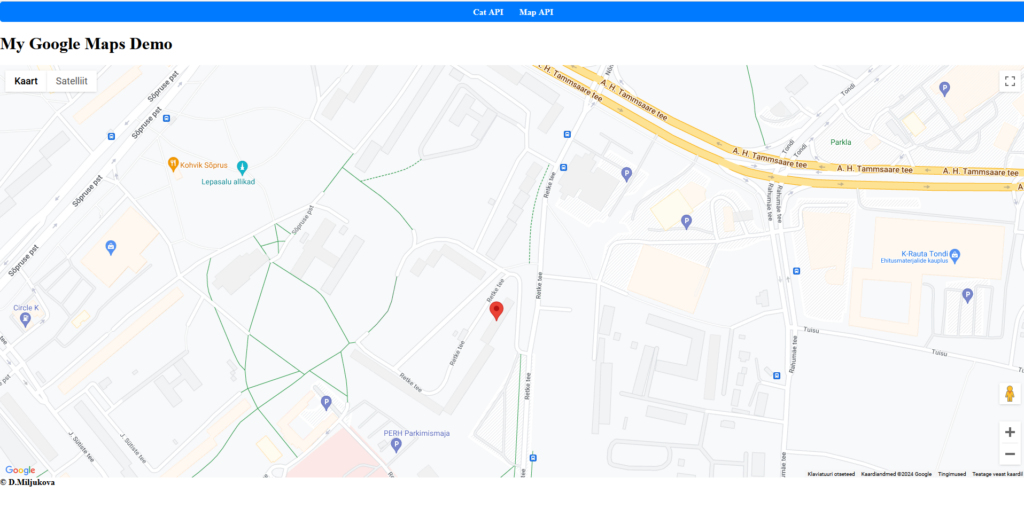
Index.html
<!DOCTYPE html>
<html lang="est">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Juhuslikud kassid</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<nav>
<ul>
<li>
<a href="../catapi/index.html">Cat API</a>
</li>
<li>
<a href="../apimap/index.html">Map API</a>
</li>
</ul>
</nav>
<div class="container">
<h1>Juhuslik kassipilt</h1>
<img id="catImage" src="" alt="Кошка" onclick="fetchCatImage()" />
</div>
<script src="script.js"></script>
</body>
<footer>
<strong>© D.Miljukova</strong>
</footer>
</html>
Style.css
body {
text-align: center;
font-family: 'Arial', sans-serif;
margin: 0;
padding: 50px;
background-color: #f0f8ff;
color: #333;
}
h1 {
font-size: 2.5em;
margin-bottom: 20px;
color: #4a4a4a;
text-shadow: 2px 2px 4px rgba(0, 0, 0, 0.2);
}
img {
width: 400px;
height: 300px;
object-fit: cover;
border: 4px solid #ff69b4;
border-radius: 15px;
cursor: pointer;
transition: transform 0.3s;
}
img:hover {
transform: scale(1.05);
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
background: white;
border-radius: 10px;
box-shadow: 0 4px 20px rgba(0, 0, 0, 0.1);
}
nav {
background-color: #007bff;
padding: 10px;
border-radius: 5px;
margin-bottom: 20px;
}
nav ul {
list-style: none;
padding: 0;
margin: 0;
display: flex;
justify-content: center;
}
nav li {
margin: 0 15px;
}
nav a {
color: white;
text-decoration: none;
font-weight: bold;
font-size: 16px;
transition: color 0.3s;
}
nav a:hover {
color: #d4d4d4;
}
Script.js
async function fetchCatImage() {
const response = await fetch('https://api.thecatapi.com/v1/images/search');
const data = await response.json();
document.getElementById('catImage').src = data[0].url;
}
window.onload = fetchCatImage;
